Optimize Images & Boost Performance of Your Statically Generated Site
Statically generated sites, or HTML sites generated using static data and templates, offer a wide variety of benefits, including much faster page load speed. If you are building high-traffic landing pages, a static website built with best practices increases conversions because the pages feel faster to the end users—and you get a lift in your search engine rankings to boot. Static site generators like Jekyll, Hugo, and Gatsby combine the speed of static sites with the scale and flexibility many companies require.
However, speed often comes at the cost of the visual experience. Creating pages that look good on screens of different sizes is harder when you can’t write any backend code. The images on your static website tend to be the largest obstacle: serving images in the right sizes to different users is key to a fast static website with visual appeal, but it’s very difficult to do using just the frontend technology.
In this article, we will cover the technical challenges of serving images on static sites, as well as ways you can overcome those problems by using Imgix to serve images of the right quality and format to your users.
Why static sites load quickly
Static sites are faster than dynamic sites for two main reasons:
- Ability to rapidly generate each page. Static sites manage this by serving the same pre-generated pages to everyone. With all of the JavaScript code already taken care of, the result is a simple and ready-to-use HTML website that loads quickly. A dynamic website, in contrast, adds latency needed to generate each page.
- Faster hosting. It is common for static sites to be deployed to a cloud storage service like Netlify or Amazon S3, and such cloud services provide fast access to their files from anywhere in the world. Some developers also choose to use a content delivery network (CDN) that places copies of the website on servers closer to the end users. The CDN speeds up the load time even further. In comparison, it’s more difficult and more expensive to place a copy of a dynamic website on 100-plus servers around the world.
Yet a typical page on a static website is full of assets—images, JavaScript, and CSS—that must be served quickly to avoid bottlenecks and retain customers. A static site isn’t built to handle dynamic assets or accommodate a responsive design, and including those components will negatively affect page performance.
Note: If you’re unsure whether images are slowing down your website and you want to establish a baseline, we recommend checking out our page weight tool.
One size doesn’t fit all
A one-size-fits-all image doesn’t, in fact, fit all devices. How do we retain the benefits of a static site without the bottlenecks that single-size images bring?
To provide responsive images while also remaining lightweight, you’ll need to serve optimized images for different browsers by creating a server-side script that generates all of the image sizes. Now, if your entire site is static and doesn’t have a backend, you likely don’t want to build and host a backend just for resizing images. Implementing such a backend can be technically complex, and storing pre-generated images in the cloud can be very costly.
How Imgix helps serve images quickly on static sites
We built the Imgix platform to serve the correct image size and format on the fly. Imgix delivers lightning-fast images without having to wait for any server-side scripts. Imgix’s on-the-fly generation also reduces your costs compared to pre-generating all image sizes ahead of time.
Using Imgix can boost a static site even more, for two reasons:
- Imgix applies intelligent compression to the images, achieving the fastest possible image download speed depending on which browser each website visitor uses.
- By default, Imgix reduces the size of even the smallest images by simplifying the color palette and removing unnecessary metadata (although you can override this behavior).
The downstream benefits of a higher-performance site are many, perhaps the most significant being that you’ll achieve higher conversion rates and provide a better user experience. Imgix is built to work with the tools and platforms you are familiar with. As a result, you can easily optimize your responsive image delivery without having to change how you build your websites.
Three ways to include Imgix images on a static site
Imgix offers several ways to include images on a static site. Let’s look at three very different ways: using regular HTML, using the react-imgix
library, and using the Jekyll library.
Using HTML
First, let’s look at how to use an img
tag in HTML without Imgix. The srcset
attribute is the secret to serving the right image for each client. Placing it inside an img
tag is straightforward:
<img
srcset="pineneedles.jpg 1x, pineneedles-2.jpg 2x, pineneedles-3.jpg 3x"
src="pineneedles.jpg"
/>
Although this allows for appropriate sizes to be delivered to the user, the service must generate and store each version of every asset, which can be rather costly. This approach isn’t sustainable with a large number of assets or when you have user-generated content. Imgix provides a simple way to integrate the srcset
without having to resize all images ahead of time: by generating the right sizes on the fly.
Using Imgix, all you need to do is use the w
and dpr
URL parameters to have your entire library generate the srcset
attributes for your images. To see this in action, we’ll use the image located at https://assets.imgix.net/unsplash/pineneedles.jpg:
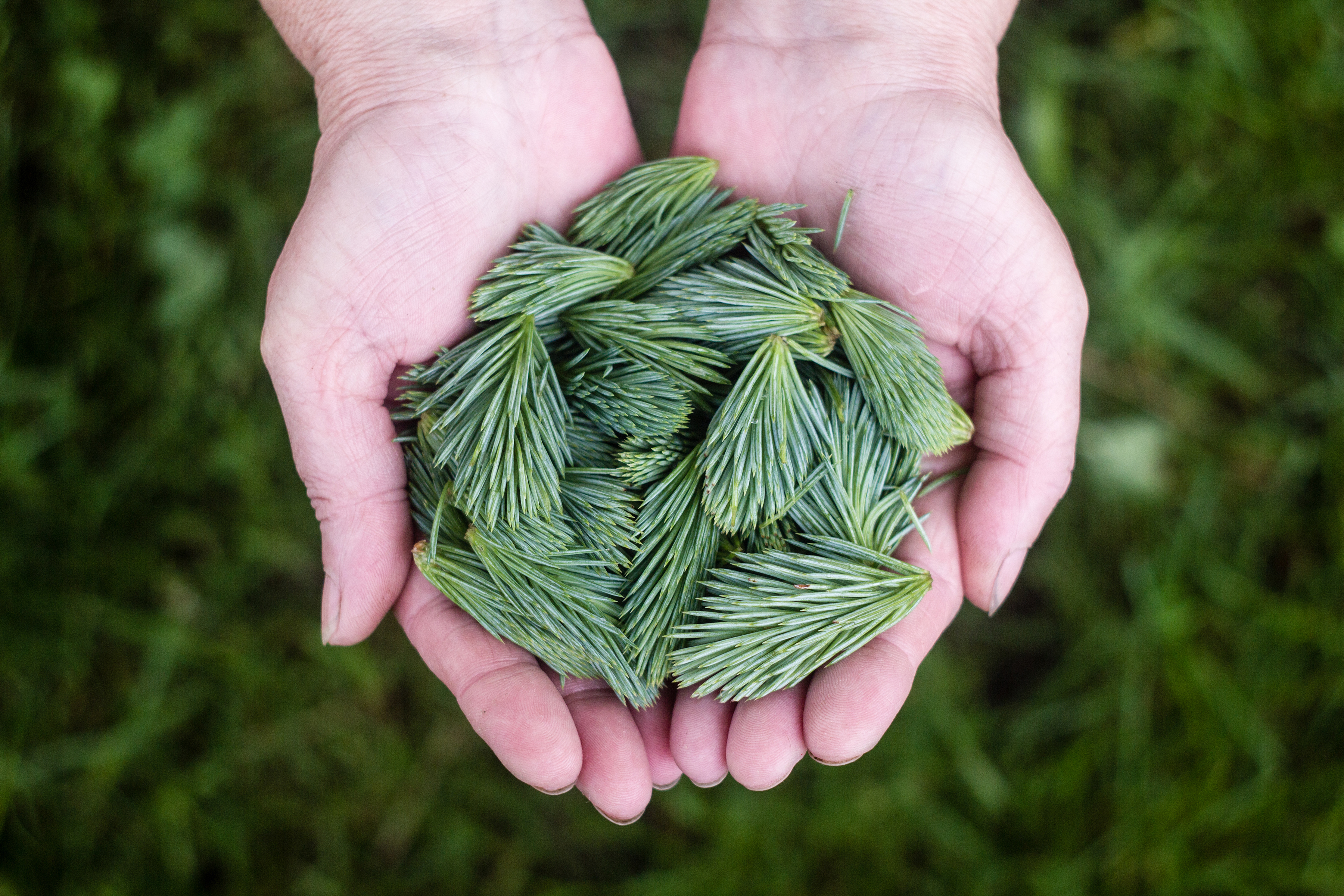
Let’s assume that our page design requires the image to be 200 pixels wide for optimal user experience. Here is how we can specify this width using the Imgix URL parameters:
<img srcset="https://assets.imgix.net/unsplash/pineneedles.jpg?w=200&dpr=1 1x,
https://assets.imgix.net/unsplash/pineneedles.jpg?w=200&dpr=2 2x,
https://assets.imgix.net/unsplash/pineneedles.jpg?w=200&dpr=3 3x"
src="https://assets.imgix.net/unsplash/pineneedles.jpg?w=200"
>
With this code, we’re able to serve the best resolution for any device by using its device-pixel-ratio (DPR) as a parameter. It’s that easy!
Using the React library
Managing the srcset
attribute for all of the different screen sizes can be difficult. But if you’re using React or any other languages we support, you’re in luck—we’ve created an Imgix library that automatically sets the srcset
attribute on your images. Each library constructs the srcset
value in a way that maximizes image size and quality while minimizing the time it takes for your customers to download the image.
The React library offers a lot of flexibility, including allowing users to leverage other elements like <img>
and <picture>
, providing ample room for creative direction. Using these HTML elements with Imgix allows you to maintain control over design features like aspect ratio or size when you need to do more than simply deliver a high-resolution image.
Let’s see how a simple child component that renders an image and a header might look:
export const Pineneedles = () => (
<div className="pineneedles">
<Imgix
sizes="calc(10% - 10px)"
src="https://assets.imgix.net/unsplash/pineneedles.jpg"
/>
<h2>These are pine needles</h2>
</div>
)
This is equivalent to the following HTML (compressed for brevity):
<img
sizes="calc(10% - 10px)"
srcset="https://assets.imgix.net/unsplash/pineneedles.jpg?auto=format&ixlib=react-9.0.2&w=100 100w,
https://assets.imgix.net/unsplash/pineneedles.jpg?auto=format&ixlib=react-9.0.2&w=116 116w,
https://assets.imgix.net/unsplash/pineneedles.jpg?auto=format&ixlib=react-9.0.2&w=134 134w,
...
https://assets.imgix.net/unsplash/pineneedles.jpg?auto=format&ixlib=react-9.0.2&w=7400 7400w,
https://assets.imgix.net/unsplash/pineneedles.jpg?auto=format&ixlib=react-9.0.2&w=8192 8192w"
src="https://assets.imgix.net/unsplash/pineneedles.jpg?auto=format&ixlib=react-9.0.2"
/>
This code uses the <Imgix/>
element from the react-imgix
library to render the image. The element uses srcset
and sizes
, allowing the browser to appropriately render the image immediately using the dimensions the user specifies.
As far as the React library goes, the above use case is just the tip of the iceberg. We can also achieve more complicated goals, like building an entire gallery by mapping images or using Background mode to render an image optimized for the background container’s natural dimensions in the DOM.
Using the Jekyll plugin
Jekyll is a popular static site generator that has its own Imgix framework. A single Jekyll Filter, imgix_url
, is responsible for handling the library’s functionality. All you need to do is pass in an image path to the variable. We will use https://assets.imgix.net
as the base URL.
Now just specify the desired image, as well as optional parameters, in an img
tag:
<img srcset="{{ '/unsplash/pineneedles.jpg' | imgix_url: w: 800, h: 600 }} 1x,
{{ '/unsplash/pineneedles.jpg' | imgix_url: w: 800, h: 600, dpr: 2 }} 2x, {{
'/unsplash/pineneedles.jpg' | imgix_url: w: 800, h: 600, dpr: 3 }} 3x" src={{
"/unsplash/pineneedles.jpg" | imgix_url: w: 800, h: 600 }} sizes="100vw" >
See this sandbox for an example of the img
tag using the pine needles image from earlier.
This is equivalent to the following HTML:
<img
srcset="
https://assets.imgix.net/unsplash/pineneedles.jpg?w=800&h=600 1x,
https://assets.imgix.net/unsplash/pineneedles.jpg?w=800&h=600&dpr=2 2x,
https://assets.imgix.net/unsplash/pineneedles.jpg?w=800&h=600&dpr=3 3x
"
src="https://assets.imgix.net/unsplash/pineneedles.jpg?w=800&h=600"
sizes="100vw"
/>
It’s as simple as that!
Conclusion
In this article, we covered the benefits of static sites as well as some common problems that come with using them. We explained how using Imgix can help you maximize the speed of your website and deliver an optimal user experience by serving all images in exactly the right sizes. We also covered three ways to get started with Imgix on your static website.
Interested in getting started? Check out how to set up imgix.